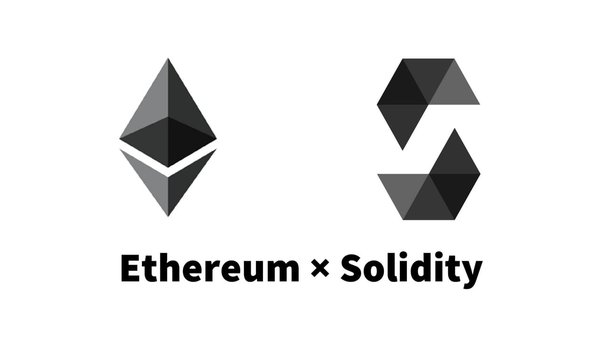
Transferring 0.0002 ETH via Smart Contract in Remix IDE #
To transfer 0.0002 ETH (which is equivalent to 200,000,000,000,000 wei) from your account (0xBD72800f108f33615cF79D74fac36F91C50D9a8C
) to another address (0xBC51107F8E0c019C0E67F3d87d382aFFA8DF0360
) using a smart contract in Remix IDE, follow the step-by-step instructions below.
Prerequisites: #
MetaMask: Ensure that you have MetaMask installed and connected to the zkEVM Testnet or any Ethereum-compatible testnet you plan to use.
Test ETH: Ensure that your account (
0xBD72800f108f33615cF79D74fac36F91C50D9a8C
) has enough Test ETH for the transaction (at least 0.01 Test ETH should be sufficient to cover gas fees).Testnet Faucet: You can request free Test ETH from the zkEVM Testnet Faucet or another Testnet faucet.
Step 1: Writing the Solidity Contract #
Create a new .sol
file in Remix and add the following simple ETH transfer contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SimpleETHTransfer {
// Event for logging the ETH transfer
event ETHTransfer(address indexed from, address indexed to, uint256 amount);
// Function to transfer ETH to a specified address
function transferETH(address payable recipient) public payable {
require(msg.value > 0, "You must send some ETH");
// Transfer the ETH to the recipient
recipient.transfer(msg.value);
// Emit an event with transfer details
emit ETHTransfer(msg.sender, recipient, msg.value);
}
// Receive ETH into the contract
receive() external payable {}
}
Step 2: Compile the Contract #
- In Remix, navigate to the Solidity Compiler tab.
- Select Solidity version 0.8.x from the dropdown menu (make sure it matches
^0.8.0
in the contract). - Click Compile to compile the contract.
- If there are no errors, the contract should successfully compile.
Step 3: Deploy the Contract #
- After compiling, switch to the Deploy & Run Transactions tab.
- Under the Environment dropdown, select Injected Web3. This will connect Remix to your MetaMask wallet (ensure MetaMask is connected to your zkEVM Testnet or desired testnet).
- You should see your MetaMask account (
0xBD72800f108f33615cF79D74fac36F91C50D9a8C
) as the default address. - Scroll down and click Deploy. MetaMask will prompt you to confirm the transaction and display the gas fees.
- Confirm the transaction in MetaMask. Wait for the contract deployment to be mined and confirmed on the zkEVM Testnet.
Step 4: Transfer 0.0002 ETH Using the Deployed Contract #
Once the contract is deployed, it will appear under the Deployed Contracts section in Remix. Now, you can use the transferETH
function to transfer ETH.
- In the Deployed Contracts section, expand the deployed
SimpleETHTransfer
contract. - Enter the recipient address (
0xBC51107F8E0c019C0E67F3d87d382aFFA8DF0360
) in the transferETH function’s input field. - In the Value (ETH) field (located above the function list in Remix), input the amount you wish to transfer in wei:
- 0.0002 ETH = 200000000000000 wei.
- Click the transferETH button to initiate the transfer.
- MetaMask will prompt you to confirm the transaction, showing the gas fees and the ETH amount. Confirm the transaction in MetaMask.
- Wait for the transaction to be mined. You can view the transaction details and logs in Remix or on the zkEVM Testnet block explorer.
Step 5: Verify the Transfer #
After the transaction is confirmed, you can verify the transfer on the zkEVM Testnet block explorer.
- Go to the zkEVM Testnet Explorer or the explorer for your chosen testnet.
- Search for the recipient address (
0xBC51107F8E0c019C0E67F3d87d382aFFA8DF0360
). - Verify that 0.0002 ETH has been received in the transaction logs.
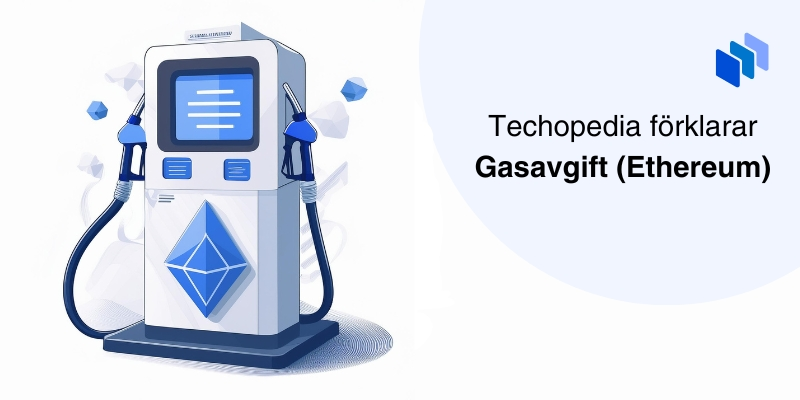
Troubleshooting Gas Errors #
If you encounter gas errors, such as "Gas estimation errored with the following message: gas required exceeds allowance", here are a few steps to resolve the issue.
Issue 1: Gas Estimation Error #
Error: "Gas required exceeds allowance."
Solution:
- This occurs when Remix or MetaMask fails to properly estimate the gas required to deploy the contract or send the transaction. You can resolve this by manually setting the Gas Limit.
Manually Set Gas Limit in MetaMask:
- When MetaMask pops up to confirm the transaction, click Edit under Gas Fee settings.
- In the Advanced settings, increase the Gas Limit manually.
- Set the Gas Limit to 1,000,000 (1 million gas units) to start with. You can adjust if needed.
Increase Gas Limit in Remix:
- In Remix, go to the Deploy & Run Transactions tab.
- Under the Gas Limit field, set the value to 1000000 or higher.
- This should prevent gas underestimation issues during contract deployment or ETH transfers.
Issue 2: Insufficient Test ETH #
Error: Not enough ETH to cover gas fees.
Solution:
- Ensure that your account (
0xBD72800f108f33615cF79D74fac36F91C50D9a8C
) has enough Test ETH.- You can request Test ETH from the zkEVM Testnet Faucet or other testnet faucets (depending on your network).
- Verify that your MetaMask wallet has at least 0.01 Test ETH to cover gas fees for deploying and transferring ETH.
Issue 3: Force Sending the Transaction #
Error: MetaMask warns that gas estimation will likely fail.
Solution:
- If you’re confident that the transaction is valid and will succeed, you can force send the transaction.
- When MetaMask displays the gas estimation error, click Yes to force send the transaction.
- This should only be done if you are sure the contract logic is correct and the transaction is expected to succeed.
Understanding ETH and Wei #
Ethereum’s native currency, ETH, is measured in wei in Solidity smart contracts. Here’s the breakdown of the conversion:
- 1 ETH = 10^18 wei
- Therefore, to transfer 0.0002 ETH, you need to provide the equivalent amount in wei:
- 0.0002 ETH = 200,000,000,000,000 wei.
Correcting for Wei in Remix #
In the Value (ETH) field of Remix, input the transfer amount in wei rather than in ETH. For example:
- To transfer 0.0002 ETH, enter 200000000000000 wei in the Value (ETH) field.
Conclusion #
By following the steps outlined above, you should be able to:
- Deploy the ETH transfer contract in Remix.
- Transfer ETH from your account to another address using the contract.
- Troubleshoot common gas issues that may occur during deployment or transfer.
- Properly handle wei values when specifying ETH amounts in Remix.
Let me know if this solves your problem or if you need any additional help!